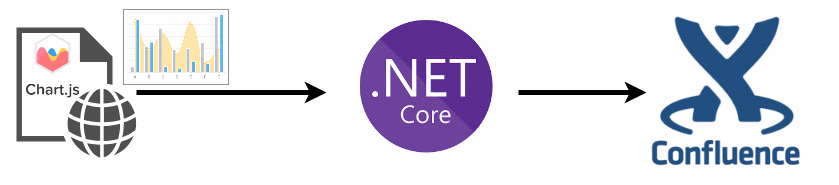
Canvas Chart ➡️ Snapshot ➡️ Manipulate ➡️ Send it to your confluence page (or elsewhere)
Today, let’s create a connection between one of your app using ChartJs + Confluence and send or synchronize a chart. The flow with Confluence server used will be with the basic authentication. In the case you want to build a real plugin/extension to Confluence, please follow the proper guide about it. In this blog post, the basic authentication is simply a mean to an end for quick prototyping.
A complete example of a standalone project can be found on Github (https://github.com/Nordes/HoNoSoFt.PushChartToConfluence.Sample).
Introduction
Like I said, we will have an application serving some charts using the Canvas (HTML5) technology. We will then resize, take a snapshot and then transmit this file to our backend server. Then it will connect to confluence, look at the existing attachments and if it exists it will update using a comment or in the case where the file does not exists, we will be posting a new file. It is nice to update the file, but why not add the file (image) to the page if it is not already there. 🙂
Proposal using a chart
Note that we’re going to add the chart, but the same flow could be used to also update some comment/text. As long as you are familiar with html syntax, you should be able to do something good.
Pre-requisites before starting
- If you already have a confluence server or follow the official installation guide from Confluence, skip to #3 (Create a small application)
- For the docker users, let’s go towards the evaluation “just because”
docker pull atlassian/confluence-server:latest
- About 700mo
- While it download, you can start by requesting a KEY
- https://my.atlassian.com/license/evaluation
- Select a Confluence server license and keep that page open until the docker image is ready.
- Start the docker image
- Command:
docker run -v $volume$:/var/atlassian/application-data/confluence --name="confluence" -d -p 8090:8090 -p 8091:8091 atlassian/confluence-server
- $volume$: C:/demo/your/confluence/home
- Open site: http://localhost:8090/
- Command:
- During installation, fetch the Server ID and input it in the evaluation page.
- Follow the instructions
- Select trial
- Get the Server ID
- Go on your atlassian page already open and paste it
- Generate your key
- Copy/paste your key in the form within the confluence page
- Click next (wait a little) and voilà
- Setup a user or two and then let’s start
- Follow the instructions
- Create a small application with ChartJs (or use the one built in the previous post )
Back-End
In the back-end, we will first be receiving the image to at least test our local upload between front-end and back-end. After, we will start integrating the Confluence connexion.
Dotnet ImagesController: Receive Images (Part 1)
Within Dotnet Core, we can receive files or forms controls using the IFormCollection. I don’t think it is common to receive such thing, but when talking about receiving files, this comes handy. The client (JavaScript) will then create a collection and send it using a multipart/form-data. In there we can find many things, however what we will only using here are the files (((IFormCollection)MyFormCollection).Files).
Let’s create a controller named ImagesController we will receive the files as stream and then copy those files to a temporary folders. If you already played around with file streaming you can probably skip this part.
[Route("api/[controller]")] [ApiController] public ImagesController : ControllerBase { // .. some constructor stuff /// <summary> /// This will save the image in your local "temp" folder. /// </summary> [HttpPost] [ProducesResponseType((int)HttpStatusCode.Created)] public async Task<IActionResult> PostAsync(IFormCollection formCollection) { var files = formCollection.Files; long size = files.Sum(f => f.Length); List<string> fileList = new List<string>(); foreach (var formFile in files) { // full path to file in temp location var filePath = Path.GetTempFileName(); if (formFile.Length > 0) { using (var stream = new FileStream(filePath, FileMode.Create)) { // Save to file... We could remove the await, have a List<Task<..>> and then do a Task.WhenAll(myList) await formFile.CopyToAsync(stream); fileList.Add(filePath); } } } // Example of processed uploaded files returning details of the new file + original request. // You shouldn't rely on (or) trust the FileName property without validation. return Ok(new { count = files.Count, size, fileList }); } }
This is named Part 1 since we will come back in that code in order to add some code to send to confluence.
Front-End
Do the snapshot
From JavaScript, we have two methods that are quite handy to take snapshot of a Canvas. The first one is “toBlob(…)” and the second is “toDataUrl(…)“. While “toBlob” is not supported everywhere, I find it more useful for the demo, otherwise feel free to use “toDataUrl” for all browsers supports except Edge and then convert that base64 into a binary data (image/png). In case you go towards the “toDataUrl” method, don’t forget that you will have to transform the data once it’s server side for something readable (binary) as an image/png.
You will see in the next sub-section how to start from a ChartJs chart which you’d like to push to your back-end having a resolution of 1200px wide. Don’t forget that if you use an adaptive screen UX, it will not be displayed as 1200px until you do a snapshot for a fraction of a second.
How does the resizing work?
As you already know, a ChartJs canvas does not resize automatically at your wishes and if you want a snapshot, you are required to hack your way. Next, we are going to have the following flow:
Interesting part in the JS, I will skip the trivial step of generating a chart since you’re I suspect that you’re already able to do so.
// Some code (I used VueJs, but other language should look alike) snapshot: function () { // Resize to desired size, bigger it is, more heavy will be the blob file. this.$refs.chartContainer.style.width = '1200px' this.chart.resize() var ctx = this // Do a snapshot this.$refs.chart.toBlob(function (blob) { // Resize back to original size ctx.$refs.chartContainer.style.width = "" ctx.chart.resize() // Prepare the form post // The file name used will be the chart title, but depending on your case, you // might want to have something more precise based on parameter (hash, encoding, something). var filename = `${ctx.chartConfig.options.title.text}.png` var data = new FormData() data.append('file', blob, filename) const config = { headers: { 'content-type': 'multipart/form-data' } } // Post the file to your backend ctx.$http.post('/api/images', data, config) // Later in the article it should become as // ctx.$http.post('/api/images/confluence/{desiredPageId}', data, config) }, "image/png", 0.95); } // Some code
As you can see, I resize to 1200px => snapshot (toBlob) => resize back to original => send the file as multipart/form-data to the api.
The file should normally be created within your temp folder and the exact location will come back through the API. It is also part of the current data contract. Now that you have that, you can consider yourself ready for the next step, which is to transfer that buffered binary data directly to confluence using their API’s.
Back-end Part 2 – Use confluence API’s
Now that we know we can receive file and save it, we now simply need to use the Confluence API’s from Atlassian. The related documentation for that is:
- How to do basic authentication with Confluence
- Confluence REST API’s description (Pretty much all you need) (for version 6.13 at time of this writing)
API’s that we’re going to use for this demo are:
- [GET] api/content/{pageId}/child/attachment?filename={formFile.FileName}&expand=version
- Search/Retrieve the existing attachment. In case it does not exists, it will sends back an empty array.
- [POST] api/content/{pageId}/child/attachment/{attachmentData.Id}/data
- Update in case of existing attachment
- [POST] api/content/{pageId}/child/attachment
- Create the attachment resource if it was not already existing
- [GET] api/content/{pageId}?expand=version,body.storage
- Retrieve the details on the current page, especially the body specified in readable/editable way (storage). The version is also mandatory when you want to update the page.
- [PUT] api/content/{pageId}
- Api used in order to update the confluence page.
Update the ImagesController to forward to Confluence
There’s maybe more code than required in the controller. The proper approach would be to use a IDataProvider injected (for UT) and then implement the provider using the IHttpClientFactory. That way, all would be testable and also it would also put the logic where it should be. However, let’s put all for now in one place and please adapt for your needs.
// some code in the controller /// <summary> /// Receive 1 or more images to be sent to Confluence server. /// </summary> /// <param name="formCollection">The form data (only files are being consumed)</param> /// <param name="pageId">The confluence page Id</param> /// <remarks> /// More details can be found at https://developer.atlassian.com/server/confluence/confluence-rest-api-examples/ /// </remarks> [HttpPost("confluence/{pageId}")] [ProducesResponseType((int)HttpStatusCode.Created)] public async Task<IActionResult> PostToConfluence(IFormCollection formCollection, int pageId) { var files = formCollection.Files; long size = files.Sum(f => f.Length); List<string> fileList = new List<string>(); var forwardAttachmentTasks = new List<Task<FileTransferResult>>(); foreach (var formFile in files) { if (formFile.Length > 0) { forwardAttachmentTasks.Add(ForwardFileToConfluence(pageId, formFile)); } } // In case we had multiple tasks at the same time. await Task.WhenAll(forwardAttachmentTasks.ToArray()).ConfigureAwait(false); await UpdatePage(pageId, forwardAttachmentTasks).ConfigureAwait(false); return StatusCode((int)HttpStatusCode.InternalServerError, new { count = files.Count, size, fileList }); } private async Task UpdatePage(int pageId, List<Task<FileTransferResult>> forwardTasks) { if (forwardTasks.Any()) { // Update the page var pageContentResult = await _confluenceHttpClient.GetAsync($"content/{pageId}?expand=version,body.storage"); var pageContentData = JsonConvert.DeserializeObject<Models.Confluence.Content.ContentStorage>(await pageContentResult.Content.ReadAsStringAsync()); string newContent = string.Empty; foreach (var sentAttachmentTask in forwardTasks) { var sentAttachment = await sentAttachmentTask; if (sentAttachment != null && sentAttachment.Results.Any()) { // Add the file if not present on the page. var fileName = sentAttachment.Results.First().Title; if (pageContentData.Body.Storage.Value.IndexOf($"<ri:attachment ri:filename=\"{fileName}\" />") == -1) { newContent += $"<h2>You've just pushed: {fileName}</h2><p><ac:image><ri:attachment ri:filename=\"{fileName}\" /></ac:image></p>"; } // Else: Nothing to do, it's already on the page somewhere. } } // Update object (camelCase mandatory, so use a proper serializer in real life scenario) var updateQuery = new { id = pageContentData.Id, title = pageContentData.Title, status = pageContentData.Status, type = pageContentData.Type, version = new { number = pageContentData.Version.Number + 1 }, body = new { storage = new { value = pageContentData.Body.Storage.Value + newContent, representation = "storage" } } }; var result = await _confluenceHttpClient.PutAsJsonAsync($"content/{pageId}", updateQuery); } } private async Task<FileTransferResult> ForwardFileToConfluence(int pageId, IFormFile formFile) { // Start getting if attachment exists var getIfAttachmentExists = _confluenceHttpClient.GetAsync($"content/{pageId}/child/attachment?filename={formFile.FileName}&expand=version").ConfigureAwait(false); // While previous request goes on, let's get the file. byte[] data; using (var br = new BinaryReader(formFile.OpenReadStream())) { data = br.ReadBytes((int)formFile.OpenReadStream().Length); } ByteArrayContent bytes = new ByteArrayContent(data); MultipartFormDataContent multipartContent = new MultipartFormDataContent(); multipartContent.Add(bytes, "file", formFile.FileName); var attachmentRequestData = await getIfAttachmentExists; if (attachmentRequestData.IsSuccessStatusCode && attachmentRequestData.StatusCode == HttpStatusCode.OK) { // Page exists and no errors... var attachmentRequestContent = await attachmentRequestData.Content.ReadAsStringAsync().ConfigureAwait(false); var attachmentData = JsonConvert.DeserializeObject<FileSearch>(attachmentRequestContent); HttpResponseMessage putAttachmentResponse; // Update existing data. if (attachmentData.Size == 1) { multipartContent.Add(new StringContent($"Automatic update/upload from TestApplication ;)."), "comment"); putAttachmentResponse = await _confluenceHttpClient.PostAsync( $"content/{pageId}/child/attachment/{attachmentData.Results.First().Id}/data", multipartContent); // Result is 1 "item" var content = await putAttachmentResponse.Content.ReadAsStringAsync().ConfigureAwait(false); var result = JsonConvert.DeserializeObject<Models.Confluence.Result>(content); return new FileTransferResult() { Results = new Models.Confluence.Result[] { result }, Size = 1 }; } else { // Create the attachment multipartContent.Add(new StringContent($"Automatic upload from TestApplication ;)."), "comment"); putAttachmentResponse = await _confluenceHttpClient.PostAsync( $"content/{pageId}/child/attachment", multipartContent); // Result is a list of item. var content = await putAttachmentResponse.Content.ReadAsStringAsync().ConfigureAwait(false); return JsonConvert.DeserializeObject<FileTransferResult>(content); } } return default(FileTransferResult); } // some code in the controller
It’s a lot of code. By reading it, it should be really easy to understand. During the file upload to Atlassian Confluence, we add a comment (updated or created) and the version gets updated. That way you could show the changes over time.
What else you could do?
- Transform the backend in order to have a provider injected
- Create UT
- Create IT
- Change the token used in order to use the official Atlassian flow for plugins
- Automate everything back-end by creating a scheduled job (not tested, but should be feasible)
- Selenium
- Image docker + chromium
- Execute the javascript using chrome Webdriver
- Send the image using a job.
- Send a SnapShot (not necessarily from Charts) while building your backend app in a pipeline.
Conclusion
Thank you for reading and I hope you have learned something today, or at least enjoyed this article.