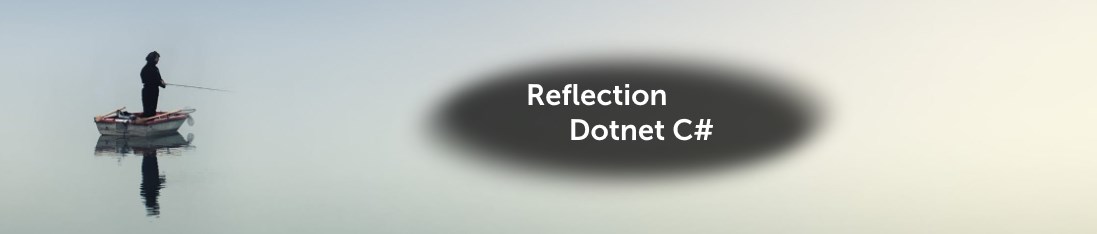
Dotnet and Reflection – Exploration By Example
Today, I will be talking about how to use reflection in order to generate code. The reflection in C# is quite strong and useful for many scenario. In this example I will simply use a dictionary created from a JSON object and then based on the “key” of that dictionary, I will create a special object if it applies, including the value. This could be useful if you receive a lot of data in from a third party, but where you would be interested only in a few keys in order to generate data somewhere in your system. Here we will not go through the performance part, but, simply know that you can alter the algorithm in order to cache the key.
What are we building?
The example will be simple and will be doing the following:
- Take JSON input
- Transform that JSON into an object (In this case, an object containing a dictionary)
- Pass through all the items from the dictionary and match the key against an enum which will be used to generate code
- Use reflection on the dictionary key enum (if it exists) and create a new special object with the dictionary value
- Print out the generated data using JSON prettify option
Building block #1 – JSON input
The JSON input will be quite simple, it will be an object containing a simple dictionary.
{ "MyDictionary": { "Key1": 1, "Key2": 2, "Key3": 3.1416 } }
Building block #2 – Basic objects structure
We now know the JSON structure, so let’s create our basic objects. Here we go a bit further than simply creating objects, we also create the Attribute which will be used during the “Reflection”.
class SampleObject { public Dictionary<string, decimal> MyDictionary { get; set; } }
public class CustomAttribute : Attribute { public string CustomValue { get; set; } public CustomAttribute(string customValue) { CustomValue = customValue; } }
enum MyDictionarySpecialKeys { [Custom("Simple Value")] Key1, [Custom("Another Simple Value")] Key3 }
class MySpecialItem { public string Special { get; set; } public string OriginalKey { get; set; } public string Value { get; set; } }
Dive in the reflection
Now that we have all the basic object, let’s talk about the Reflection. I will not repeat what you can find on the MSDN, but rather show you directly a possible usage. The Reflection, as the name indicate, can go and read through the assembly and see how the objects are made and give you useful information. Recently, I used it in order to take a JSON configuration (appsettings section) and then generate code to be executed in the background based on that. I will not explain further that application.
Let’s begin, we will be taking our “Attribute” from the enum value and use the value provided. Then from that, instead of simply returning a simple string, let’s return a new Object. In this implementation, I will be using an extension method for simplicity. The same could be done using a simple method.
public static class CustomAttributeExtension { internal static MySpecialItem GenerateEvent(this MyDictionarySpecialKeys myKey, decimal val) { // Get the member from the Enum var memberInfo = typeof(MyDictionarySpecialKeys) .GetMember(myKey.ToString()) .FirstOrDefault(); // If the enum member exists, then let's get the attribute if (memberInfo != null) { var attr = (CustomAttribute)memberInfo .GetCustomAttributes(typeof(CustomAttribute), false) .FirstOrDefault(); if (attr == null) { // Should skip, if not exists return null; } // If enum contains the attribute let's create an object return new MySpecialItem { Special = attr.CustomValue, OriginalKey = myKey.ToString(), Value = val.ToString(CultureInfo.InvariantCulture) }; } // Should skip return null; } }
Let’s put all together in a program
If we take all of what we’ve done so far and put this into a program. We will have a JSON having 3 items in a dictionary. However, that dictionary will have only two entries mapped on the strongly typed enum. From that enum, the two enum members will be decorated with a custom attribute giving a special extra meaning to the value.
That put into code will give you something like this:
public class Program { public static void Main() { var jsonInputSample = "{ \"MyDictionary\": { \"Key1\": 1, \"Key2\": 2, \"Key3\": 3.1416 } }"; Console.WriteLine($"Input: {jsonInputSample}"); var deserializedObject = JsonConvert.DeserializeObject<SampleObject>(jsonInputSample); var generatedSpecialItems = new List<MySpecialItem>(); Console.WriteLine(); Console.WriteLine("Content of [MyDictionary]:"); foreach (var dictionaryItem in deserializedObject.MyDictionary) { Console.WriteLine($"\tDictionary [Key: {dictionaryItem.Key}] [Value: {dictionaryItem.Value}]"); if (Enum.TryParse(dictionaryItem.Key, true, out MyDictionarySpecialKeys specialKey) && Enum.IsDefined(typeof(MyDictionarySpecialKeys), specialKey)) { var specialItem = specialKey.GenerateEvent(dictionaryItem.Value); if (specialItem != null) { generatedSpecialItems.Add(specialItem); } } } Console.WriteLine(); Console.WriteLine("Result (Could be special items to be inserted in a DB):"); Console.WriteLine("-----------------------------------------"); Console.WriteLine(JsonConvert.SerializeObject(generatedSpecialItems, Formatting.Indented)); Console.WriteLine("-----------------------------------------"); Console.WriteLine("Completed"); // Console.ReadKey(); // If you wish to wait when you run from Visual Studio and not command line. } }
And the output result will be:
Input: { "MyDictionary": { "Key1": 1, "Key2": 2, "Key3": 3.1416 } } Content of [MyDictionary]: Dictionary [Key: Key1] [Value: 1] Dictionary [Key: Key2] [Value: 2] Dictionary [Key: Key3] [Value: 3.1416] Result (Events to be created): ----------------------------------------- [ { "Special": "Simple Value", "OriginalKey": "Key1", "Value": "1" }, { "Special": "Another Simple Value", "OriginalKey": "Key3", "Value": "3.1416" } ] ----------------------------------------- Completed